Configuring predictions
As detailed in the previous section, ShipupETA has defaults that work well for simple scenarios. If you have more complex scenarios such as multiple warehouses or international shipping, you need to configure ShipupETA properly to receive sound predictions.
Origin and destination addresses
The origin and destination addresses are the most important parameters when computing a prediction. They have the most impact on the accuracy of delivery estimates.
For this reason, it is important to correctly specify those.
There are two ways to specify addresses: using raw address objects or using warehouses.
Using raw address objects
As detailed in the API Reference, one way to specify addresses is to specify addresses for both the init
and fetchPredictions
methods.
// Specifying addresses on the `init` method will declare global, default addresses
ShipupETA.init({
publicApiKey: 'XXX',
defaultOriginAddress: {
country_code: 'US',
state_code: 'CA'
},
defaultDestinationAddress: {
country_code: 'US',
state_code: 'NY'
}
});
// These defaults can be overridden in each call to `fetchPredictions`
ShipupETA.fetchPredictions({
origin: {
country_code: 'US',
state_code: 'TS'
}
// The destination address will still be the default US-NY
});
⚠️ Make sure to always specify a valid iso 3166-1 alpha 2 format for country codes.
Using warehouses (recommended)
The recommended way to specify addresses is by specifying the warehouse where the delivery will be shipped from.
This is the default behavior when no addresses are specified: we will fall back on the address of the default warehouse configured in the Shipup app for both the origin and the destination address.
If you configured multiple warehouses, you can specify manually which one should be used with the origin.warehouse_merchant_id
property. That property only affects the origin address.
As an example, if we configured a default warehouse with id warehouse_default
and a second one with id warehouse_2
:
ShipupETA.init({ publicApiKey: 'XXX' }); // We don't specify any default address
// Will use address from warehouse_default for both origin and destination
ShipupETA.fetchPredictions();
// Will use address from warehouse_2 for both origin and destination
ShipupETA.fetchPredictions({
origin: {
warehouse_merchant_id: 'warehouse_2'
}
});
Warehouses and raw addresses can be combined when the origin and destination countries are not the same:
ShipupETA.init({ publicApiKey: 'XXX' }); // We don't specify any default address
// Will use address from warehouse_2 for origin and France for destination
ShipupETA.fetchPredictions({
origin: {
warehouse_merchant_id: 'warehouse_2'
},
destination: {
country_code: 'FR'
}
});
If both an origin.country_code
and anorigin.warehouse_merchant_id
are specified, the warehouse id will take priority.
In summary, the whole address logic is described by the two diagrams below:
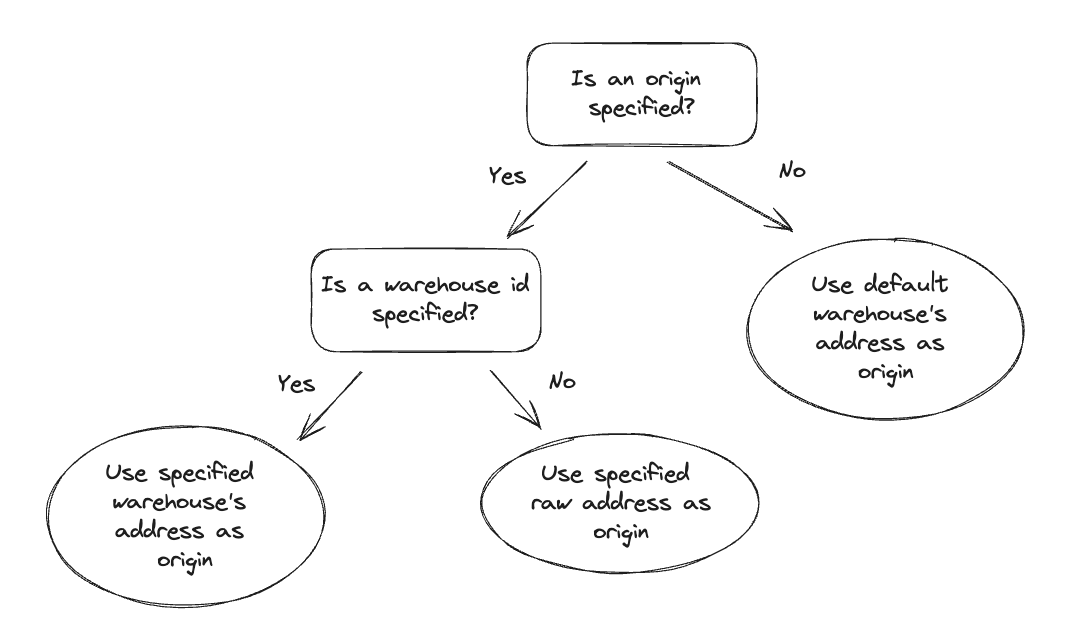
Deciding which origin address to use
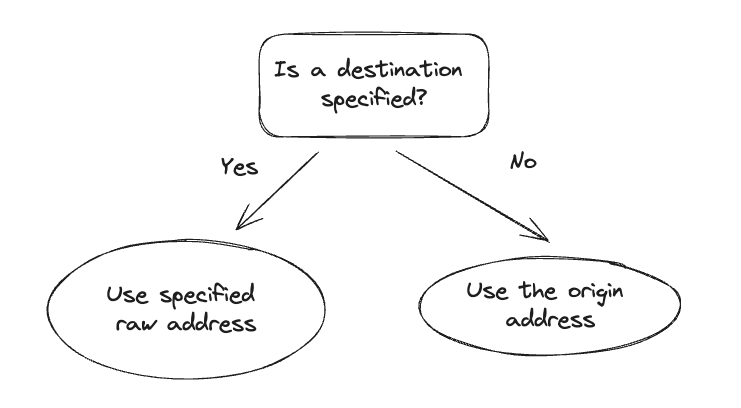
Deciding which destination address to use
Other advantages of using warehouses
By specifying warehouses you will make sure that we use the correct warehouse settings to estimate an order's shipping date. For example, we will use:
- the open/closed days of the warehouse
- the global or per-carrier cutoff-time in that warehouse
A good delivery estimate starts with a good shipping date estimate!
Filtering carriers
By default, calling fetchPredictions()
returns an estimated delivery for each carrier service enabled on your account.
You can filter the carriers and carrier services to use by specifying a carriers
option when calling fetchPredictions
:
ShipupETA.fetchPredictions({
carriers: [
{
code: 'dhl',
service_code: 'base'
},
{
code: 'royal_mail',
service_code: 'royal_mail_uk_tracked_24'
}
]
}
You can find in our documentation the list of carrier codes and service codes
Order date and shipping date
By default, calling fetchPredictions
returns an estimated delivery for an order placed at the current time. This means that the algorithm will:
- first compute a shipping date, i.e. when the order that is placed at the current time will be shipped
- then compute a delivery date based on that shipping date
In some specific cases, you may need to specify your own order or shipping date (f.i. to manually say there will be a 5 days delay for out-of-stock products, pre-orders products). Also, if you have different preparation time per product and depending on time of year, you can adjust the order processing days to override the warehouse's. You can do so by using the order.ordered_at
, order.merchant_shipping_date
or order.order_processing_time
properties:
ShipupETA.fetchPredictions({
order: {
ordered_at: '2024-12-20T12:00:00.000'
}
});
ShipupETA.fetchPredictions({
order: {
// Will skip any shipping time calculation
// and generate a prediction as if the order is shipped at the specified date
merchant_shipping_date: '2024-04-22'
}
});
ShipupETA.fetchPredictions({
order: {
// Will add 2 working days to the order datetime
order_processing_time: 2
}
});
Updated 8 months ago